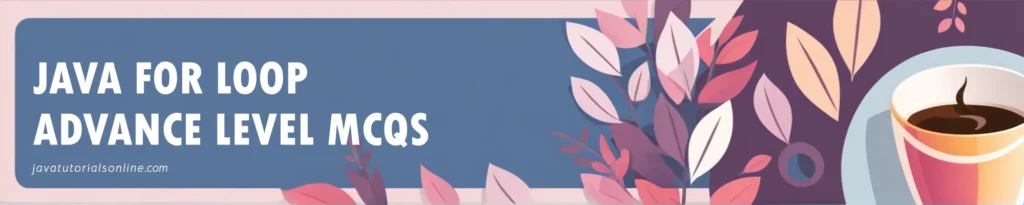
Java For Loops are essential for handling iterations efficiently. If you’ve been following our series on Java For Loops, this advanced-level set will take your knowledge to the next level. Practicing Java For Loop Advanced MCQs can be incredibly beneficial for checking your fundamental knowledge and improving your problem-solving abilities.
Don’t forget to check out our other sets for different difficulty levels:
- Java For Loop Beginner-Level MCQs [Click here]
- Java For Loop Intermediate-Level MCQs [Click here]
For Loop MCQ Questions (Hard)
Ready to test your knowledge? Let’s dive into some multiple-choice questions to reinforce your understanding of advanced Java For Loops.
What is the output of the following code?
int i = 0;
for (System.out.print(i + " "); i < 3; i++) {
System.out.print(i + " "); }
View Answer
Correct Answer: A
The first print statement happens before the loop starts, printing 0. The loop runs from i = 0 to i = 2, printing each value. So, the output is 0 1 2.
What will be the output of the following code?
for (int i = 0; i < 3; i++) {
if (i == 2) {
break;
}
System.out.print(i + " "); }
View Answer
Correct Answer: A
The loop runs from i = 0 to i = 2, but the `break` statement stops the loop when i equals 2. Thus, only 0 and 1 are printed before the break.
What is the output of this nested loop?
for (int i = 1; i <= 2; i++) {
for (int j = 1; j <= 2; j++) {
System.out.print(i * j + " ");
}
}
View Answer
Correct Answer: A
The outer loop iterates i from 1 to 2. The inner loop multiplies i by j (1 and 2). Hence, the output is 1 2 2 4.
What will be the output of the following loop?
int i = 5;
for (; i < 10; i++) {
System.out.print(i + " "); }
View Answer
Correct Answer: D
The loop starts with i = 5 and increments i until i < 10. Therefore, the output is 5 6 7 8 9.
What is the output of the following code involving nested loops?
for (int i = 1; i <= 2; i++) {
for (int j = 1; j <= 2; j++) {
System.out.print(i * j + " ");
}
}
View Answer
Correct Answer: D
The outer loop runs with i = 1 and 2. For each i, the inner loop runs j = 1 and 2. Therefore, the multiplication gives 1 2 2 4.
What will be the output of the following code with an increment in the loop?
int i = 0;
for (; i < 5; i++) {
if (i == 3) break;
System.out.print(i + " "); }
View Answer
Correct Answer: D
The loop prints 0, 1, 2 before the condition `i == 3` triggers the `break` statement, stopping the loop.
What is the output of the following code with a step increment of 2?
for (int i = 0; i < 5; i += 2) {
System.out.print(i + " "); }
View Answer
Correct Answer: C
The loop starts at 0 and increments by 2 each time, printing 0, 2, 4.
What will be the output of the following code using an enhanced for-each loop?
int[] arr = {1, 2, 3};
for (int num : arr) {
System.out.print(num + " "); }
View Answer
Correct Answer: A
The enhanced for loop iterates through the array elements in order, printing 1 2 3.
What is the output of the following code when the loop modifies the index inside the loop body?
for (int i = 0; i < 5; i++) {
System.out.print(i + " ");
i++; }
View Answer
Correct Answer: A
The loop starts at i = 0, prints it, then increments i twice, resulting in 0 2 4.
What will be the output of the following code with a decrement in the loop?
for (int i = 5; i > 0; i--) {
System.out.print(i + " "); }
View Answer
Correct Answer: A
The loop decrements from 5 to 1, printing 5 4 3 2 1.
What is the output of the following nested loop with break?
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
if (j == 2) break;
System.out.print(i + " ");
}
}
View Answer
Correct Answer: A
The inner loop breaks when j reaches 2, and prints `i` twice for each iteration of the outer loop.
What is the output of this loop that updates the counter inside the loop?
int sum = 0;
for (int i = 0; i < 4; i++) {
sum += i;
i++;
}
System.out.print(sum);
View Answer
Correct Answer: D
The loop adds 0, 2 to sum (since i is incremented inside the loop), so the sum is 6.
What is the output of this infinite loop with a break condition?
for (;;) {
System.out.print("Looping ");
break; }
View Answer
Correct Answer: A
The loop runs once, prints "Looping", and then breaks, stopping the loop.
What will be the output of the following code using an external loop control variable?
int i = 0;
for (i = 0; i < 5; i++) {
System.out.print(i + " "); }
View Answer
Correct Answer: C
The loop runs from i = 0 to i = 4, printing 0 1 2 3 4.
What is the output of this code with a continue statement?
for (int i = 1; i <= 5; i++) {
if (i == 3) continue;
System.out.print(i + " "); }
View Answer
Correct Answer: A
The `continue` statement skips when i equals 3, so the output is 1 2 4 5.
What is the output of the following code with a conditional print inside?
for (int i = 1; i < 4; i++) {
if (i % 2 == 0)
System.out.print(i + " "); }
View Answer
Correct Answer: B
The loop prints only even numbers, so the output is 2.
What will be the output of the following code snippet with a variable change inside the loop?
int x = 1;
for (int i = 1; i <= 5; i++) {
x *= i;
System.out.print(x + " "); }
View Answer
Correct Answer: A
The loop multiplies x by i each time, resulting in 1 2 6 24 120.
What is the output of the following code with a skip pattern in the loop?
for (int i = 1; i <= 5; i++) {
if (i == 4) continue;
System.out.print(i + " "); }
View Answer
Correct Answer: A
The `continue` statement skips when i equals 4, so the output is 1 2 3 5.
What will be the output of this code where the loop runs conditionally?
for (int i = 1; i <= 3; i++) {
if (i % 2 != 0)
System.out.print(i + " "); }
View Answer
Correct Answer: C
The loop prints only odd numbers, so the output is 1 3.
What is the output of the following code involving a nested for loop?
for (int i = 1; i <= 2; i++) {
for (int j = 1; j <= 2; j++) {
System.out.print(i * j + " ");
}
}
View Answer
Correct Answer: C
The loop prints the product of i and j, resulting in 1 2 2 4.
Leave a Reply