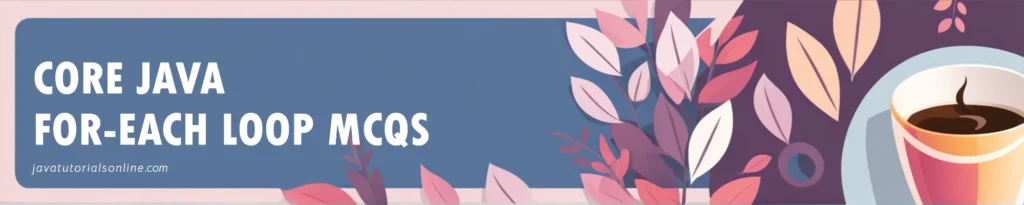
Test your understanding of Java for-each loop MCQs! Solve the following question to practice how this loop simplifies iterating over arrays and collections, enhancing code readability and efficiency.
The Java for-each loop is a simplified way to iterate over arrays or collections without using an index. It automatically loops through each element, making the code cleaner and easier to read.
for (Type element : collection) {
// Process element
}
To dive deeper into the for-each
loop, check out the official Java documentation.
What is the main purpose of the 'for-each' loop in Java?
View Answer
Correct Answer: C
The 'for-each' loop is designed to simplify the iteration process for arrays and collections by removing the need for explicit indexing.
Which of the following data structures cannot be used with a 'for-each' loop?
View Answer
Correct Answer: D
A 'for-each' loop cannot directly iterate over a Map because it is not an Iterable. However, it can iterate over the entrySet(), keySet(), or values().
What is the output of the following code?
int[] numbers = {1, 2, 3, 4};
for (int num : numbers) {
System.out.print(num + " " );
}
View Answer
Correct Answer: C
The 'for-each' loop iterates through the array, printing each element in order separated by a space.
Which statement about the 'for-each' loop is true?
View Answer
Correct Answer: B
The loop variable in a 'for-each' loop receives a copy of each element, so it cannot directly modify the original elements in the collection or array.
What is the output of the following code?
String[] words = {"Java", "Spring", "Hibernate"};
for (String word : words) {
word = "Updated";
}
System.out.println(words[0]);
View Answer
Correct Answer: A
In a 'for-each' loop, the loop variable 'word' is a copy of the array element, so modifying it does not affect the original array.
Can a 'for-each' loop be used to iterate over primitive arrays?
View Answer
Correct Answer: C
A 'for-each' loop works with all primitive arrays, such as int[], char[], double[], etc.
Which of the following causes a compilation error in a 'for-each' loop?
View Answer
Correct Answer: D
A 'for-each' loop cannot iterate over an uninitialized collection because it does not point to a valid Iterable object.
What will happen if you try to use a 'for-each' loop with a 'Map' directly?
View Answer
Correct Answer: D
A 'for-each' loop cannot iterate over a Map directly. However, you can iterate over its entrySet(), keySet(), or values().
Which of the following can be used as the loop variable in a 'for-each' loop for a 'List<Integer>'?
View Answer
Correct Answer: C
The loop variable can be of type Integer (matching the list type) or Object (since Integer is an Object). Autoboxing allows int to work as well.
What is the output of the following code?
List<String> list = Arrays.asList("A", "B", "C");
for (String s : list) {
System.out.print(s + "-");
}
View Answer
Correct Answer: B
The loop iterates over the list and appends '-' to each element, resulting in the output 'A-B-C-'.
What will happen if a 'for' loop has an empty body?
for (int i = 0; i < 5; i++);
System.out.println("Done");
View Answer
Correct Answer: C
The semicolon after the 'for' statement creates an empty loop body. The loop executes 5 times, then prints 'Done'.
What is the output of the following nested 'for' loop?
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= i; j++) {
System.out.print(j + " ");
}
}
View Answer
Correct Answer: C
The outer loop controls the rows, and the inner loop prints numbers from 1 to 'i' in each iteration. The output is '1 1 2 1 2 3'.
What is a potential pitfall of using a 'for' loop with floating-point variables?
View Answer
Correct Answer: B
Floating-point precision issues may cause the loop condition to never become false, leading to infinite loops in some cases.
Which code snippet will generate the sequence: 10, 8, 6, 4, 2?
View Answer
Correct Answer: C
The correct code snippet starts with i = 10 and continues as long as i >= 2, decrementing i by 2 in each iteration. The values are printed on each loop, producing the desired sequence.
What is the output of the following 'for' loop?
for (int i = 0; i < 5; i++) {
if (i == 3) {
continue;
}
System.out.print(i + " ");
}
View Answer
Correct Answer: C
The 'continue' statement skips the iteration when 'i == 3', so '3' is not printed.
What happens if the update statement in a 'for' loop is empty?
for (int i = 0; i < 5; ) {
System.out.println(i++);
}
View Answer
Correct Answer: D
The update logic 'i++' is placed inside the loop body instead of the update section, so the loop runs correctly and prints numbers from '0 1 2 3 4'.
What will be the output of the following code?
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 3; j++) {
if (i == j) break;
System.out.print(i + "," + j + " ");
}
}
View Answer
Correct Answer: A
The `break` exits the inner loop when `i == j`, so pairs where `i` equals `j` are skipped. The resulting output is '1,2 1,3 2,3 3,2'.
What is the behavior of the following loop?
for (int i = 1; i > 0; i++) {
if (i == 10) break;
System.out.print(i + " ");
}
View Answer
Correct Answer: A
The loop increments `i` until it reaches 10 and then exits using `break`. It prints numbers 1 to 9 before breaking.
What happens if a 'for' loop's condition is always true?
for (int i = 0; ; i++) {
if (i == 3) break;
System.out.print(i + " ");
}
View Answer
Correct Answer: A
The condition is always true, but the `break` statement ensures the loop exits when `i == 3`. The output is '0 1 2'.
What is the output of the following code?
int[] nums = {1, 2, 3};
for (int i = 0; i < nums.length; i++) {
for (int j = i; j < nums.length; j++) {
System.out.print(nums[j] + " ");
}
}
View Answer
Correct Answer: A
The outer loop starts at each index, and the inner loop prints elements from the current index to the end of the array. The output is '1 2 3 2 3 3'.
Leave a Reply