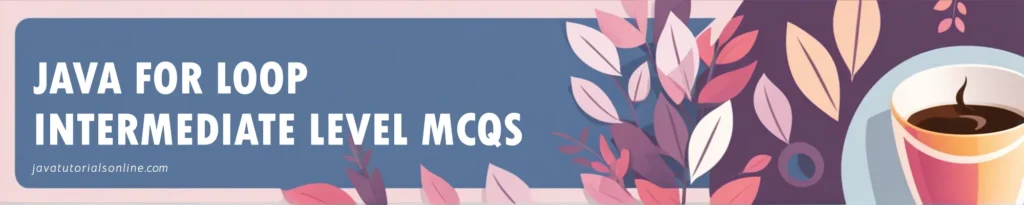
Java For Loops are essential for handling iterations efficiently.This intermediate-level set will help you bridge the gap between basic and advanced concepts. Practicing Java For Loop Intermediate-Level MCQs can improve your understanding and coding skills.
Don’t forget to check out our other sets for different difficulty levels:
- Java For Loop Beginner-Level MCQs [Click here]
- Java For Loop Advanced-Level MCQs [Click here]
For more intermediate Java topics, you can also explore additional resources on Java official documentation.
For Loop MCQ Questions (Medium)
Ready to test your knowledge? Let’s dive into some multiple-choice questions to reinforce your understanding of intermediate Java For Loops.
What will be the output of the following code?
for (int i = 1; i <= 5; i++) {
System.out.print(i * i + " ");
}
View Answer
Correct Answer: C
The loop prints the square of each number from 1 to 5, which results in: 1 4 9 16 25.
Which code snippet will print the numbers 5 10 15 20?
View Answer
Correct Answer: B
Option 2 iterates from 5 to 20 in steps of 5, producing the correct sequence: 5 10 15 20.
What is the output of this code?
for (int i = 1; i <= 4; i++) {
for (int j = 1; j <= 2; j++) {
System.out.print(i * j + " ");
}
}
View Answer
Correct Answer: B
The nested loop multiplies i and j, producing the sequence: 1 2 2 4 3 6 4 8.
Which code snippet produces the same result?
for (int i = 1; i <= 3; i++) {
System.out.print(i + " ");
}
View Answer
Correct Answer: C
Option 3 produces the same output by iterating from 0 to 2 and adding 1 to each iteration value.
What will be the output of this code?
for (int i = 10; i > 0; i -= 2) {
System.out.print(i + " ");
}
View Answer
Correct Answer: C
The loop iterates with a decrement of 2, printing the sequence: 10 8 6 4 2.
Which code will output '1 2 4 8 16'?
View Answer
Correct Answer: A
Option 1 uses `Math.pow` to print the powers of 2: 1 2 4 8 16.
What will this code output?
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 3; j++) {
System.out.print(i * j + " ");
}
}
View Answer
Correct Answer: B
The nested loop multiplies i and j, producing: 1 2 3 2 4 6 3 6 9.
Which loop will print 'A B C D E'?
View Answer
Correct Answer: C
Option 1 correctly loops from 'A' to 'E' and prints each letter: A B C D E.
What will be the output of this loop?
for (int i = 1; i <= 5; i++) {
System.out.print(i + " ");
if (i == 3) break;
}
View Answer
Correct Answer: A
The loop breaks after printing '3', so the output is: 1 2 3.
Which code snippet will print '5 10 15 20'?
View Answer
Correct Answer: C
Option 2 loops from 1 to 4 and prints multiples of 5, resulting in: 5 10 15 20.
What will be the output of this code?
for (int i = 1; i <= 5; i++) {
System.out.print(i + " ");
if (i == 3) continue;
}
View Answer
Correct Answer: B
The `continue` statement skips the 3rd iteration, printing: 1 2 4 5.
Which code will print '1 1 2 4 8'?
View Answer
Correct Answer: C
Option 1 prints the powers of 2: 1 2 4 8.
What will be the output of this loop?
for (int i = 5; i > 0; i--) {
System.out.print(i + " ");
}
View Answer
Correct Answer: A
The loop runs backwards, printing: 5 4 3 2 1.
Which code will output '1 2 3 4 5'?
View Answer
Correct Answer: B
Both option 1 and 2 will print the numbers: 1 2 3 4 5.
Which code will produce '2 4 6 8 10'?
View Answer
Correct Answer: B
Option 1 correctly iterates from 2 to 10 in steps of 2, printing the even numbers.
What will be the output of this code?
for (int i = 1; i <= 5; i++) {
System.out.print(i + " ");
if (i == 3) break;
}
View Answer
Correct Answer: C
The loop breaks at the 3rd iteration, so the output is: 1 2 3.
What is the output of the following code?
for (int i = 1; i <= 5; i++) {
System.out.print(i * i + " ");
}
View Answer
Correct Answer: C
The loop prints the square of each number from 1 to 5: 1 4 9 16 25.
Which code will print '1 3 5 7 9'?
View Answer
Correct Answer: A
Option 1 correctly calculates the odd numbers between 1 and 9: 1 3 5 7 9.
What will be the output of this code?
for (int i = 2; i <= 6; i += 2) {
for (int j = 1; j <= 3; j++) {
System.out.print(i * j + " ");
}
}
View Answer
Correct Answer: A
The nested loop multiplies i and j, producing: 2 4 6 4 8 12 6 12 18.
Which loop will print '1 2 3 4 5 6 7 8 9 10'?
View Answer
Correct Answer: A
Option 1 will correctly print the numbers from 1 to 10.
Leave a Reply