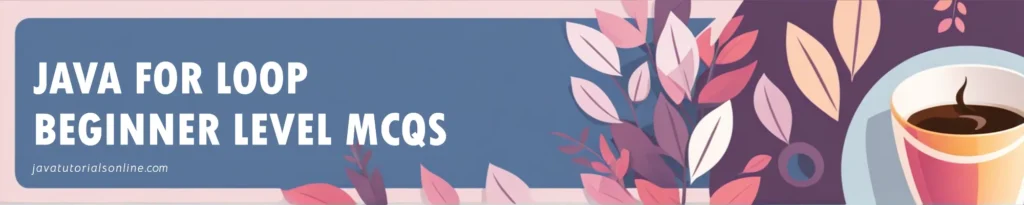
Java For Loops are essential for handling iterations efficiently. A for loop is a control flow statement that allows code to be executed repeatedly based on a given condition. It consists of three parts: initialization, condition, and update. Here’s the basic syntax of a for loop in Java:
for (initialization; condition; update) {
// code to be executed
}
This beginner-level set will help you practice Java For Loop Beginner-Level MCQs to build your foundational knowledge and gain confidence in your coding skills.
Don’t forget to check out our other sets for different difficulty levels:
- Java For Loop Intermediate-Level MCQs [Click here]
- Java For Loop Advanced-Level MCQs [Click here]
For more beginner Java topics, you can also explore additional resources on Java’s official documentation.
For Loop MCQ Questions (Easy)
Now, let’s put your knowledge to the test with some multiple-choice questions designed to reinforce your understanding of basic Java For Loops.
What is the output of the following loop?
for (int i = 1; i <= 3; i++) {
System.out.print(i + " ");
}
View Answer
Correct Answer: B
The loop starts from 1 and runs until 3, printing each value: 1 2 3.
What will the following code print?
for (int i = 2; i <= 6; i += 2) {
System.out.print(i + " ");
}
View Answer
Correct Answer: A
The loop increments by 2, starting at 2 and ending at 6, printing: 2 4 6.
Which code produces the output: 5 10 15 20?
View Answer
Correct Answer: B
Option 1 multiplies the loop index by 5 and prints the result: 5 10 15 20.
What will be the output of this nested loop?
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 2; j++) {
System.out.print(i * j + " ");
}
}
View Answer
Correct Answer: A
The outer loop runs 3 times, and the inner loop runs 2 times each time, producing: 1 2 2 4 3 6.
What is the output of the following code?
for (int i = 0; i < 5; i++) {
System.out.print(i + " ");
}
View Answer
Correct Answer: B
The loop starts from 0 and runs until 4, printing: 0 1 2 3 4.
Which of the following code snippets will print the numbers 10 20 30?
View Answer
Correct Answer: C
Option 2 runs the loop with increments of 10, printing: 10 20 30.
What will be the output of this loop?
for (int i = 1; i <= 5; i++) {
if (i % 2 == 0) {
System.out.print(i + " ");
}
}
View Answer
Correct Answer: A
The loop prints only even numbers, so the output is: 2 4.
Which of the following loops will print 'Hello' 3 times?
View Answer
Correct Answer: C
Option 1 runs the loop 3 times, printing 'Hello' each time.
What is the output of the following code?
for (int i = 1; i <= 4; i++) {
System.out.print(i * 2 + " ");
}
View Answer
Correct Answer: C
The loop multiplies each index by 2, printing: 2 4 6 8.
What will the following code output?
for (int i = 3; i >= 1; i--) {
System.out.print(i + " ");
}
View Answer
Correct Answer: C
The loop starts from 3 and decrements until it reaches 1, printing: 3 2 1.
What is the output of this code snippet?
for (int i = 1; i <= 5; i++) {
System.out.print(i + " ");
if (i == 3) break;
}
View Answer
Correct Answer: B
The loop breaks when i equals 3, so the output is: 1 2 3.
What is the output of the following nested loops?
for (int i = 1; i <= 2; i++) {
for (int j = 1; j <= 2; j++) {
System.out.print(i * j + " ");
}
}
View Answer
Correct Answer: C
The outer loop runs twice, and for each iteration, the inner loop runs twice, printing: 1 2 2 4.
What will the following code print?
for (int i = 0; i < 5; i++) {
System.out.print((i + 1) + " ");
}
View Answer
Correct Answer: B
The loop prints i + 1, so the output is: 1 2 3 4 5.
What is the output of this loop?
for (int i = 2; i <= 6; i += 2) {
System.out.print(i * 2 + " ");
}
View Answer
Correct Answer: A
The loop multiplies each index by 2, printing: 4 8 12.
Which code snippet will produce the output: 1 2 3 4 5?
View Answer
Correct Answer: B
Option 1 prints the numbers from 1 to 5: 1 2 3 4 5.
What will this code print?
for (int i = 1; i <= 4; i++) {
if (i % 2 == 1) {
System.out.print(i + " ");
}
}
View Answer
Correct Answer: B
The loop prints only odd numbers, so the output is: 1 3.
Which of the following loops prints 'Java' 4 times?
View Answer
Correct Answer: C
Option 1 prints 'Java' 4 times.
What will the following code print?
for (int i = 2; i <= 8; i += 2) {
System.out.print(i + " ");
}
View Answer
Correct Answer: C
The loop runs in steps of 2, printing: 2 4 6 8.
What will be the output of this loop?
for (int i = 5; i >= 1; i--) {
System.out.print(i + " ");
}
View Answer
Correct Answer: B
The loop prints the numbers in descending order: 5 4 3 2 1.
Which code produces the output: 10 20 30 40?
View Answer
Correct Answer: B
Option 1 prints the multiples of 10: 10 20 30 40.
Leave a Reply